
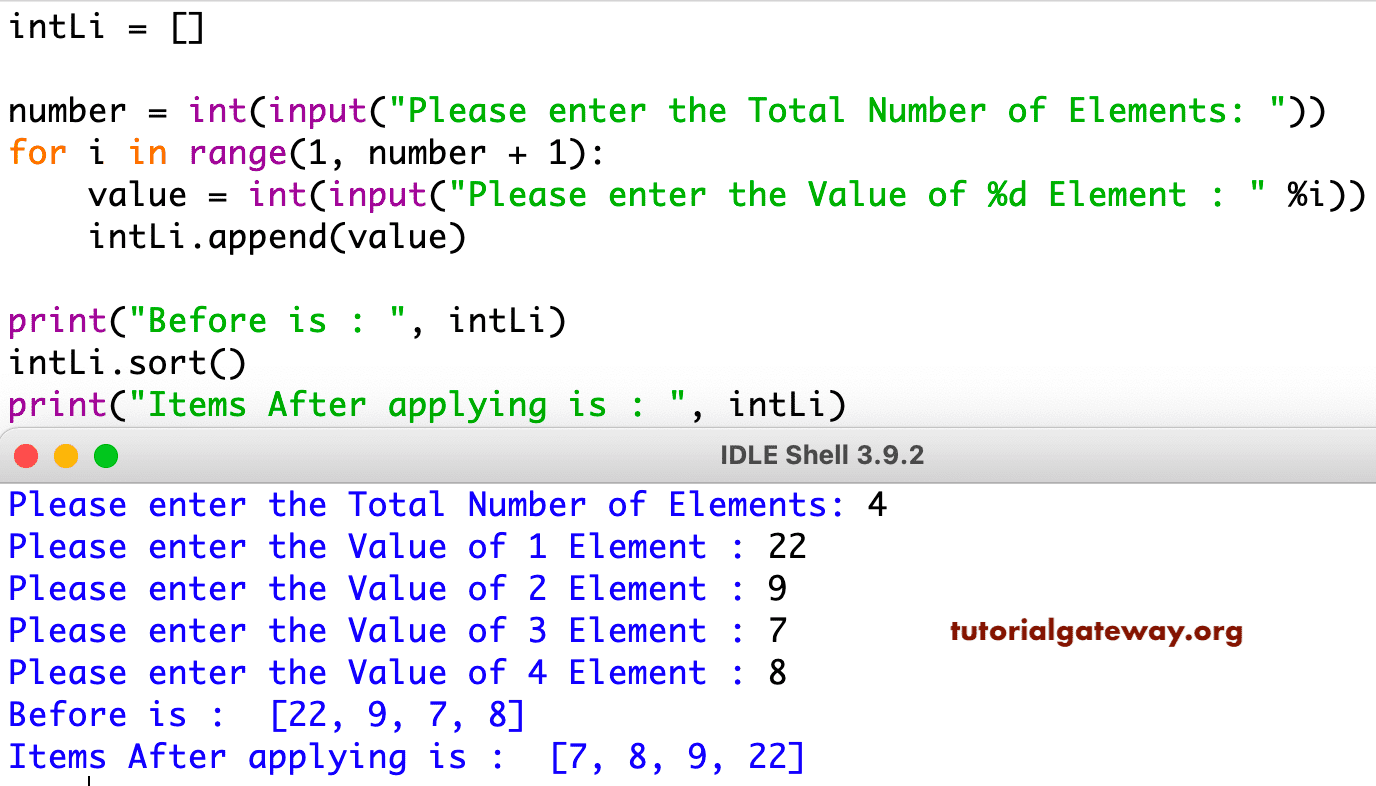
Print(sorted(salary_info.values(), reverse=True)) # sort the dictionary values in descending order Pass reverse=True to the sorted() function. 1 Answer Sorted by: 17 if you have numbers as values, you can use this: stats.sort (keylambda x: (-x 'K', -x 'B', x 'A', x 'Z')) For general values: stats.sort (keylambda x: (x 'A', x 'Z')) stats. In this approach first, we will sort all the keys in the dictionary, and then we will perform sorting on the list values. You can also sort the values in descending order.
Python sort list of dictionaries on key how to#
In particular, you're going to learn how to sort a list of dictionaries in. The values are sorted in ascending order. You'll learn the ins and outs of the sorting function in Python. When we pass the dictionary into the sorted () function by default it sorts the keys of the dictionary and returns them as a list. It can be used to sort dictionaries by key in ascending order or descending order. Use the dictionary values() function to get the dictionary values and then apply the sorted() function to sort the returned values. We can sort the dictionary by key using a sorted () function in Python. You can similarly use the sorted() function to sort a dictionary’s values. The keys in the output are sorted in descending order. Print(sorted(salary_info.keys(), reverse=True)) # sort the dictionary keys in descending order Pass reverse=True to the sorted() function. You can also sort the keys in descending order. You can see that the keys are present in sorted order. Use the dictionary keys() function to get the dictionary keys and then apply the sorted() function to sort the returned keys. You can use Python built-in sorted() function to sort the keys of a dictionary. In this tutorial, we will look at how to sort a dictionary’s keys and values separately and not inside the dictionary itself. Note that from Python 3.7 and above, dictionaries are ordered by their keys by default. OrderedDict can be imported from the collections module in Python. If you want an inherent order to a dictionary’s items, use OrderedDict instead.
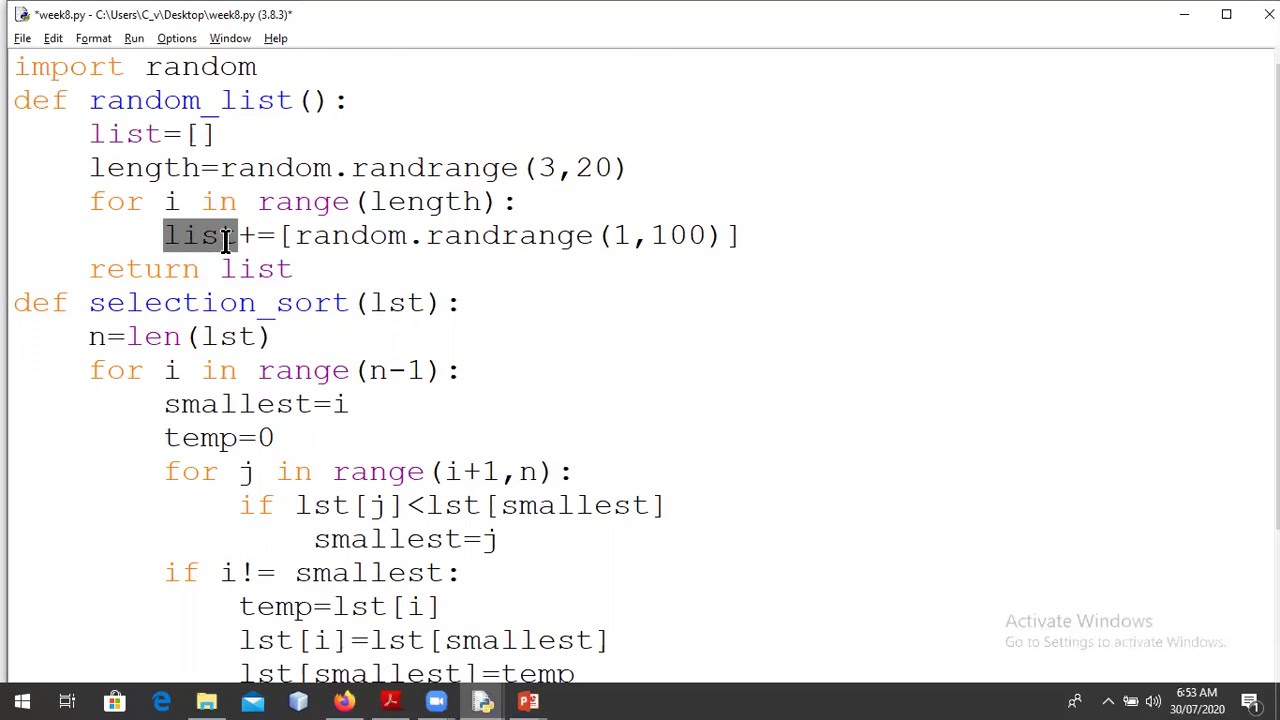
That is, there’s no inherent order to the keys or values present in a dictionary. Normal Python dictionaries are unordered. In this article, I have explained how to sort a list of dictionaries by value in ascending/descending order using Pyhton sorted(), sort(), lambda function, and itemgetter() functions with examples.In this tutorial, we will look at how to sort the keys and values of a Python dictionary with the help of some examples. This constructor keeps track of the insertion order of key-value pairs in the dictionary. Dictionary elements should be enclosed with ] Python dictionaries can also be created using the dict () constructor by simply providing a list or tuple of comma-separated key-value pairs. Each element in the dictionary is in the form of key:value pairs. # Example 8: Sort a list of dictionaries having the same value for multiple keysĪ Python dictionary is a collection that is unordered, mutable, and does not allow duplicates. Print(sorted(dict_list, key=emgetter('calories'))) # Example 7: Sort list of dictionaries without key existence Print(sorted(dict_list, key=emgetter('fruit_name'))) # Example 6: Set emgetter() as key and sort Print(sorted(dict_list, key=lambda x: x.get('calories', 95))) # Example 5: Sort list of dictionaries using sorted() & get() Print(sorted(dict_list, key=lambda x: x.get('calories'))) # Example 4: Sort list of dictionaries using sorted() & get() # Example 3: Sort list of dictionaries when key doesn't exist Print(sorted(dict_list, key=lambda x: x, reverse = True)) # Example 2: Sort list of dictionaries in descending order Print(sorted(dict_list, key=lambda x: x)) By the way, the sorted() function sorts a dictionary by keys by default. # Example 1: Sort list of dictionaries using sorted() & lambda To sort a dictionary by keys in Python, call the sorted() function on dictionary items. To sort a list of dictionaries by a value of the dictionary, you can use the sorted() function and specify the key parameter to be a lambda function that.

If you are in a hurry, below are some quick examples of how to sort a list of dictionaries by value. Quick Examples of Sort a List of Dictionaries by Value In this article, I will explain how to sort a list of dictionaries by value in ascending/descending order using Pyhton sorted() function and this function is also used to sort dictionary by value and sort dictionary by Key in Python. This function sorts iterable objects like lists, tuples, sets, and dictionaries. Using sorted() function we can sort a list of dictionaries by value in ascending order or descending order. Sorting is always a useful utility in everyday programming. We can sort a list of dictionaries by value using sorted() or sort() function in Python.
